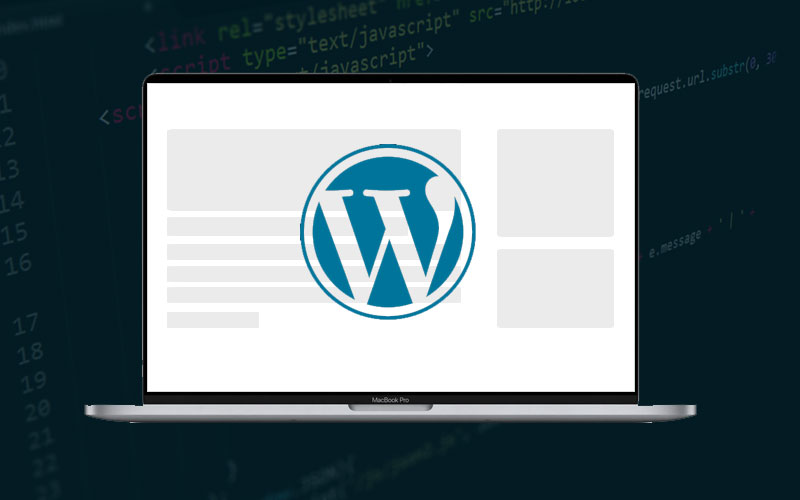
To install WordPress on a remote Linux server using SSH, you can use a Python script with the paramiko
library, which allows for SSH connections, and execute the necessary commands remotely.
Steps to follow:
- Connect to the server via SSH.
- Update the system and install required packages.
- Download and configure WordPress.
- Set up a database for WordPress.
- Configure the Apache/Nginx web server and permissions.
Here’s a Python script using paramiko
to automate this process:
Python Script:
import paramiko
import time
# Define server and credentials
hostname = 'your_server_ip'
username = 'your_ssh_user'
password = 'your_ssh_password' # Or use SSH key for better security
# Commands to be executed on the remote server
commands = [
"sudo apt update && sudo apt upgrade -y", # Update system
"sudo apt install apache2 mysql-server php libapache2-mod-php php-mysql -y", # Install Apache, MySQL, PHP
"sudo systemctl start apache2", # Start Apache server
"sudo systemctl enable apache2", # Enable Apache to start on boot
"sudo mysql_secure_installation", # Secure MySQL installation (interactive)
# Create MySQL Database for WordPress
"""sudo mysql -e "CREATE DATABASE wordpress DEFAULT CHARACTER SET utf8 COLLATE utf8_unicode_ci;" """,
"""sudo mysql -e "CREATE USER 'wordpressuser'@'localhost' IDENTIFIED BY 'password';" """,
"""sudo mysql -e "GRANT ALL ON wordpress.* TO 'wordpressuser'@'localhost';" """,
"""sudo mysql -e "FLUSH PRIVILEGES;" """,
# Download WordPress
"wget https://wordpress.org/latest.tar.gz",
"tar -xvzf latest.tar.gz",
"sudo mv wordpress /var/www/html/",
# Set permissions
"sudo chown -R www-data:www-data /var/www/html/wordpress",
"sudo chmod -R 755 /var/www/html/wordpress",
# Set up Apache configuration for WordPress
"""sudo bash -c 'cat << EOF > /etc/apache2/sites-available/wordpress.conf
<VirtualHost *:80>
ServerAdmin admin@example.com
DocumentRoot /var/www/html/wordpress
ServerName example.com
<Directory /var/www/html/wordpress>
AllowOverride All
</Directory>
ErrorLog ${APACHE_LOG_DIR}/error.log
CustomLog ${APACHE_LOG_DIR}/access.log combined
</VirtualHost>
EOF'""",
"sudo a2ensite wordpress.conf", # Enable the new site
"sudo a2enmod rewrite", # Enable URL rewriting
"sudo systemctl restart apache2", # Restart Apache
]
def run_ssh_commands(hostname, username, password, commands):
try:
# Initialize SSH client
client = paramiko.SSHClient()
client.set_missing_host_key_policy(paramiko.AutoAddPolicy())
# Connect to the server
print(f"Connecting to {hostname}...")
client.connect(hostname, username=username, password=password)
# Execute each command
for command in commands:
print(f"Executing: {command}")
stdin, stdout, stderr = client.exec_command(command)
time.sleep(2) # Give time for command to execute
output = stdout.read().decode()
error = stderr.read().decode()
if output:
print(output)
if error:
print(f"Error: {error}")
# Close connection
client.close()
print("Completed installation.")
except Exception as e:
print(f"An error occurred: {e}")
# Run the script
run_ssh_commands(hostname, username, password, commands)
Explanation:
- SSH Connection: Uses
paramiko
to establish an SSH connection. - Update & Install: The script updates the server, installs Apache, MySQL, and PHP.
- MySQL Setup: Creates a MySQL database and user for WordPress.
- Download WordPress: Downloads and moves WordPress to the Apache web directory.
- Configure Apache: Sets up an Apache virtual host for WordPress.
- Permissions: Configures appropriate ownership and permissions for WordPress files.
Requirements:
- Install
paramiko
using pip:bashCopy codepip install paramiko
- Modify the script with your server’s IP, SSH username, and password.
« How to choose the best IVR solution for your business Webhook Explained for Beginners: Everything You Need to Know »