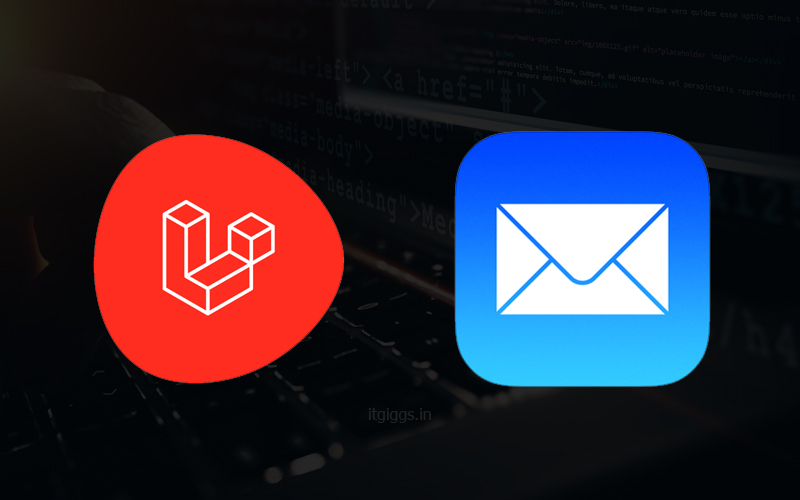
#1 Setup the SMTP configurations in ENV file
MAIL_MAILER=smtp
MAIL_HOST=mail.example.com
MAIL_PORT=465
MAIL_USERNAME=admin@example.com
MAIL_PASSWORD=SMTP_Password
MAIL_ENCRYPTION=ssl
MAIL_FROM_ADDRESS=admin@example.com
MAIL_FROM_NAME="${APP_NAME}"
Please Note – Don’t forget to update the host and mail from address
#2 Create mail using the artisan command
Run the following command to make a mail instance in laravel
php artisan make:mail MyFancyEmail
#3 Create the Email Template
Just like views, we need to create a blade template file for email too.
Create a file named MyFancyEmail.blade.php in resources/views/email/ folder
<!DOCTYPE html>
<html>
<head>
<title>Hello from Laravel</title>
</head>
<body>
<h1>{{ $details['title'] }}</h1>
<p>{{ $details['contents'] }}</p>
<p>Thank you</p>
</body>
</html>
#4 Create a Controller to send Email
Create a controller by running the following command
php artisan make:controller EmailController
This command will generate a file app/Http/Controllers/EmailController.php
add the following code in this file –
/* add this in header */
use App\Mail\MyFancyEmail;
/**************************/
class EmailController extends Controller
{
public function enquiry(Request $request)
{
$mail_content = [
'title' => 'New Email from Laravel',
'body' => 'Silence is Golden'
];
Mail::to('someone@example.com')->send(new MyFancyEmail($mail_content));
}
}