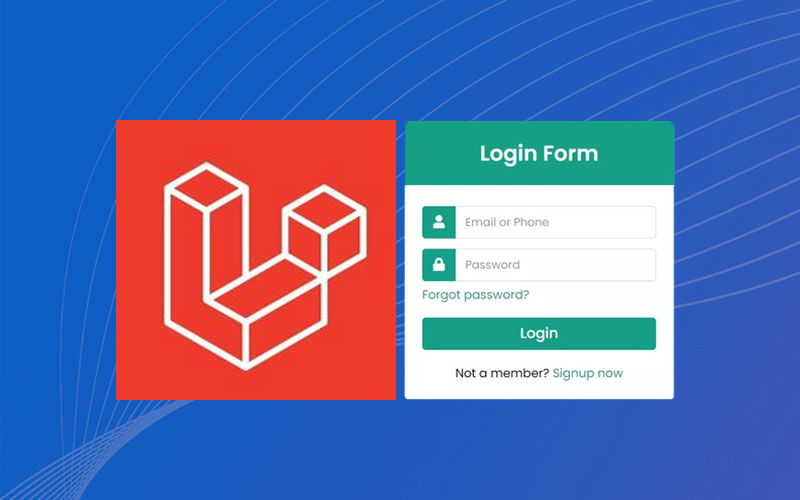
What is Laravel?
Laravel is one of the most popular PHP Frameworks based on MVC & is used in a variety of websites including Portals & CMS etc.
Getting Started
Install Composer
Composer is basically a PHP dependency manager similar to npm in the case of Node.js applications.
Create Laravel Project
After installing Composer, you can create a new laravel project by simply running the commands below –
composer create-project laravel/laravel example-app
cd example-app
php artisan serve
Now, you can test your installation by visiting http://localhost:8000 in the browser of your choice.
Add Authentication to Your Application
composer require laravel/ui:^2.4
php artisan ui vue --auth
Setup Database Connection
Go to the .env file in the root folder and configure the database credentials as shown below-
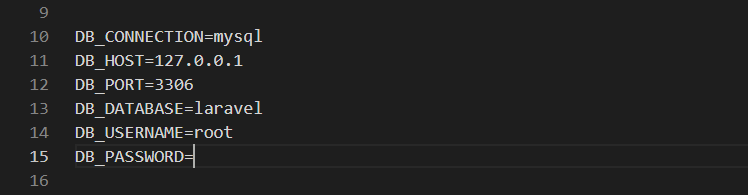
Now you’re all set.
Just run the below command from terminal and verify your installation-
php artisan serve
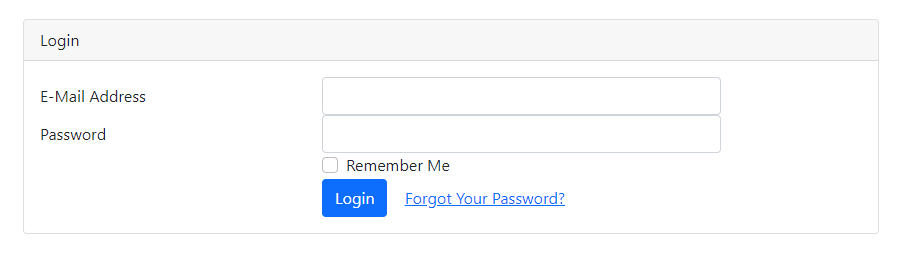

Restricted Access in Laravel
The ultimate goal of Authentication is to restrict users from reaching certain pages, based on their role.
So, to do that we need a guy called Middleware. What middleware does is act like a gatekeeper, checking each http request & if the current user is allowed to open it or not.
How to make pages accessible to Logged in Users only?
If you simply want to allow logged-in users to access a page, You only need to pass the middleware in the routes as mentioned below-
Route::get('/restricted-page',[SomeController::class, 'index'])->middleware(['auth']);
Because laravel-auth provides you with a default middleware “auth”, you just applied on the route above. What if you need to apply user-role based restriction? let’s find out-
Multi-Role Based Authentication in Laravel
Step 1 – Add a column for user role in the database table.
By Default, laravel-auth doesn’t make a column for user role, so we need to make that first.
Go to the database/migrations directory & edit the file similar to —-create_users_table.php
public function up()
{
Schema::create('users', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->string('email')->unique();
$table->timestamp('email_verified_at')->nullable();
$table->string('password');
$table->string('role')->default('user');
$table->rememberToken();
$table->timestamps();
});
}
Note – We’ve taken the default user role as “user”.
Step 2 – Create a new Middleware
Use the below command to create a middleware –
php artisan make:middleware Role
Step 3 – Apply the Restrictions
Go to App/Http/Middlewares & update the Role.php file as shown below-
public function handle(Request $request, Closure $next)
{
if (Auth::check()) {
if (Auth::user()->role == 'admin') { // if the current role is Administrator
return $next($request);
}
}
abort(403, "Cannot access to restricted page");
}
It should look like this –
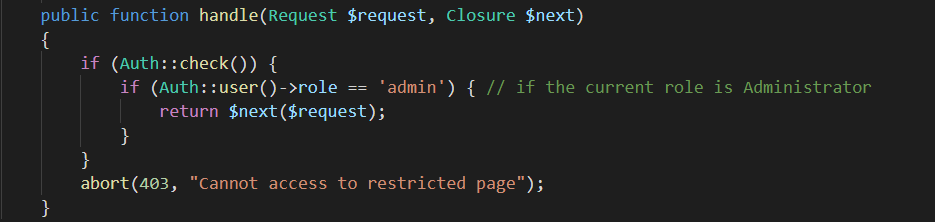
Step 4 – Configure Middleware in Kernel.php file
protected $routeMiddleware = [
.........
'role' => \App\Http\Middleware\Role::class,
..........
];
It should look like this –
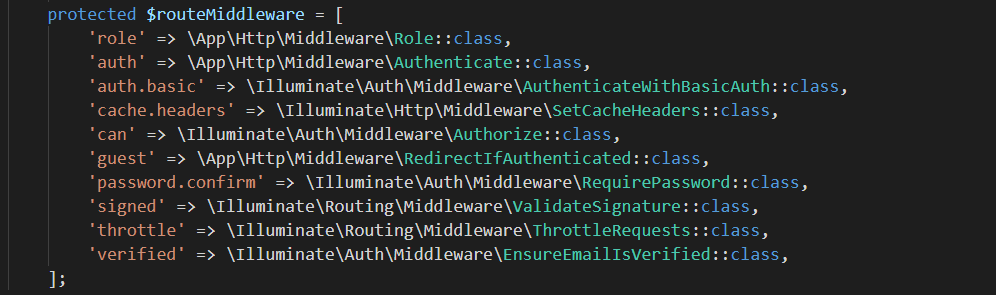
Step 5 – Apply the middleware on routes
Now, you can apply the middleware just created in the same way we did earlier –
Route::get('/restricted-page',[SomeController::class, 'index'])->middleware(['role']);
And we’re Done!
You can now test it by opening the restricted URL in the browser.
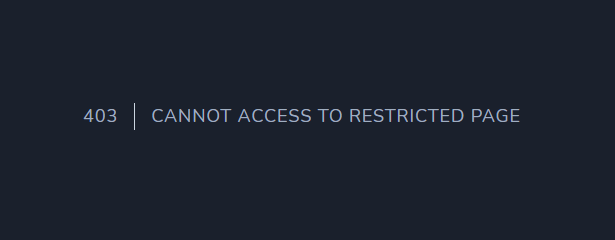