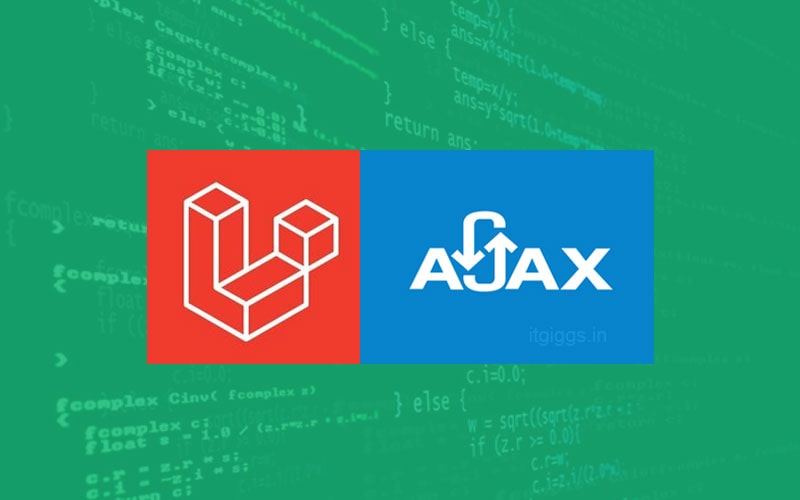
In this step-by-step tutorial, we’ll learn how to check if the user already exists in laravel via AJAX.
Check if User Exists in Laravel using AJAX
Create the View
Create a new file checkemail.blade.php and add the following code in it-
<form method="POST" action="{{ route('register') }}" id="registerform">
@csrf
<label for="name" class=" text-md-right">{{ __('Name') }}</label>
<input id="name" type="text" class="form-control @error('name') is-invalid @enderror" name="name" value="{{ old('name') }}" required autocomplete="name" autofocus>
@error('name')
<span class="invalid-feedback" role="alert">
<strong>{{ $message }}</strong>
</span>
@enderror
<label for="email" class=" text-md-right">{{ __('E-Mail Address') }}</label>
<input id="email" type="email" class="form-control @error('email') is-invalid @enderror" name="keyword" value="{{ old('email') }}" required autocomplete="email" onfocusout="checkemail()">
<div id="emailresponse"></div>
@error('email')
<span class="invalid-feedback" role="alert">
<strong>{{ $message }}</strong>
</span>
@enderror
<label for="password" class=" text-md-right">{{ __('Password') }}</label>
<input id="password" type="password" class="form-control @error('password') is-invalid @enderror " name="password" required autocomplete="new-password">
@error('password')
<span class="invalid-feedback" role="alert">
<strong>{{ $message }}</strong>
</span>
@enderror
<label for="password-confirm" class=" text-md-right">{{ __('Confirm Password') }}</label>
<input id="password-confirm" type="password" class="form-control mb-2" name="password_confirmation" required autocomplete="new-password">
<button type="submit" class="btn btn-primary">
{{ __('Register') }}
</button>
</form>
<script>
function checkemail(){
$('#emailresponse').text("Checking Availability");
var csrf = $('meta[name="csrf-token"]').attr('content');
var ENDPOINT = "{{ url('/') }}";
var data = $("#registerform").serialize();
$.ajaxSetup({
headers: {
'X-CSRF-TOKEN': $('meta[name="csrf-token"]').attr('content')
}
});
$.ajax({
'url': ENDPOINT + "/check-email",
'datatype': "html",
'type': "post",
'_token': csrf,
'data' : data,
})
.done(function (response) {
console.log(response);
$('#emailresponse').html(response);
});
}
</script>
Create the Controller
To create a controller, run the following command in terminal –
php artisan make:controller ajaxController
This will create a file ajaxController.php in App/http/Controllers
add the following code in the header of file –
#1 Include User Model
use App\Models\User;
#2 Add the function to check if User Exists
public function checkemail(Request $request){
$email = $request->keyword;
if(!empty($email)){
if (User::where('email', '=', $email)->exists()) {
echo "<small class='text-danger'>User Already Exists</small>";
}else{
echo "<small class='text-success'>Email Good to Go</small>";
}
}
}
Define the Routes
Go to web.php inside Routes directory
Include the Controller – First we need to include the controller you created in previous step. To do so add the following code in the header of web.php file
use App\Http\Controllers\ajaxController;
Define the Route – Now, we need to specify the route for our POST requests. Just add the following code in the web.php file below header.
Route::post('/check-email', [ajaxController::class, 'checkemail']);