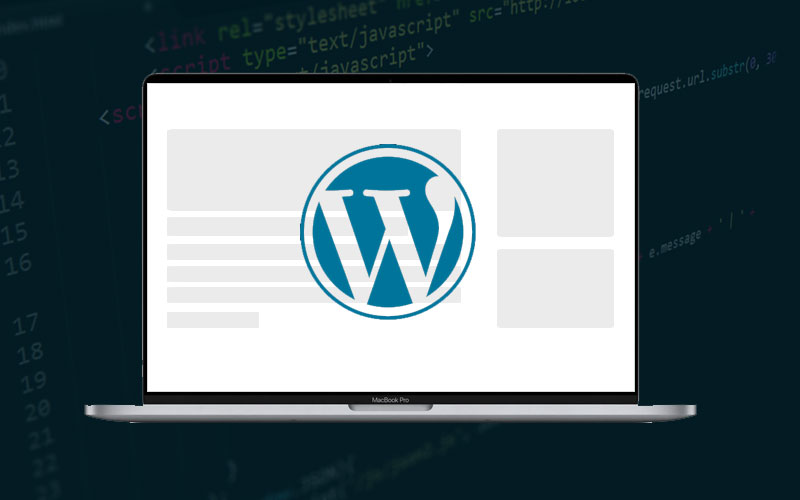
Introduction
Widgets are certain components in WordPress which you can drag and place in any widget sidebar.
Function to Make your Own Widget
Code Example to make a Recent Posts Widget
Different Type of Widgets you can create in WordPress
- Recent Posts Widget
- Recent Comments Widget
- Categories Widget
- Gallery Widget
- Contact form widget
- Newsletter Widget.
- Social Links Widget.
Let’s Get Started
1. Make Widget Class
class recent_posts_widget extends WP_Widget {
//widget code here
}
2. Construct Function
//widget constructor function
function __construct() {
$widget_options = array (
'classname' => 'class recent_posts_widget',
'description' => 'Widget to display latest posts.'
);
parent::__construct( 'my_custom_widget', 'Recent Posts', $widget_options );
}
3. Create the Form
//function to output the widget form
function form( $instance ) {
$title = ! empty( $instance['title'] ) ? $instance['title'] : '';
$postcount = ! empty( $instance['postcount'] ) ? $instance['postcount'] : 'Select No. of Posts';
?>
<p>
<label for="<?php echo $this->get_field_id( 'title'); ?>">Title:</label>
<input class="widefat" type="text" id="<?php echo $this->get_field_id( 'title' ); ?>" name="<?php echo $this->get_field_name( 'title' ); ?>" value="<?php echo esc_attr( $title ); ?>" /></p>
<p>
<label for="<?php echo $this->get_field_id( 'postcount'); ?>">Select No. of Posts</label>
<input class="widefat" type="number" id="<?php echo $this->get_field_id( 'postcount' ); ?>" name="<?php echo $this->get_field_name( 'postcount' ); ?>" value="<?php echo esc_attr( $postcount ); ?>" /></p>
<?php }
4. Save the Widget
//function to define the data saved by the widget
function update( $new_instance, $old_instance ) {
$instance = $old_instance;
$instance['title'] = strip_tags( $new_instance['title'] );
$instance['postcount'] = strip_tags( $new_instance['postcount'] );
return $instance;
}
5. Show the widget
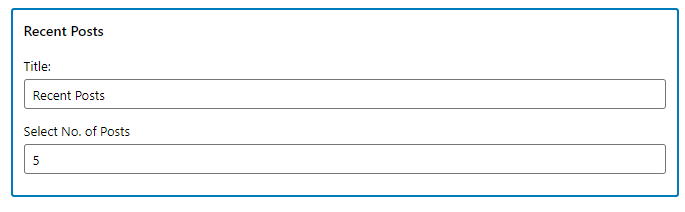
//function to display the widget in the site
function widget( $args, $instance ) {
//define variables
$title = apply_filters( 'widget_title', $instance['title'] );
$postcount = $instance['postcount'];
//output code
echo $args['before_widget']; ?>
<div class="widget-area">
<?php if ( ! empty( $title ) ) {
echo $args['before_title'] . $title . $args['after_title'];
};
//widget output starts
$query_args = array(
'post_type' => 'post',
'status' => 'publish',
'posts_per_page' => $postcount
);
// the query
$the_query = new WP_Query( $query_args );
if ( $the_query->have_posts() ) :
while ( $the_query->have_posts() ) : $the_query->the_post(); ?>
<p ><a href="" class="text-dark"><?php the_title(); ?></a></p>
<?php endwhile;endif; ?>
</div>
<?php
//widget output ends
echo $args['after_widget'];
}
Complete Code to Make a Recent Posts Widget in WordPress
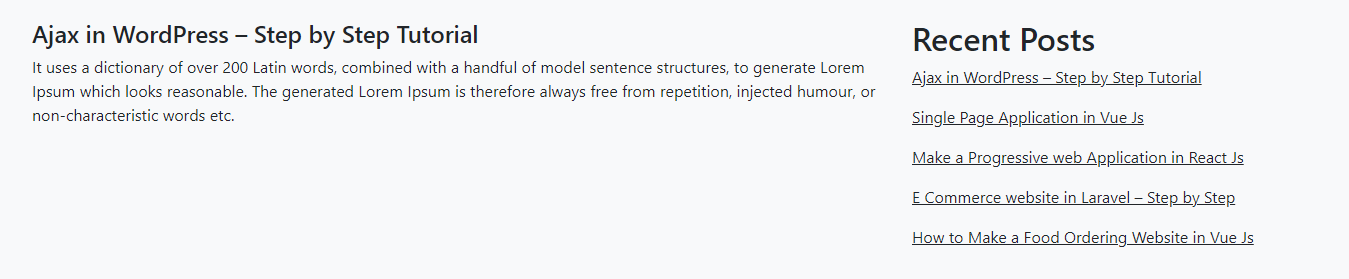
Paste this code into your theme’s functions.php file
Register a Sidebar to Place Your widget
Paste this code into your theme’s functions.php file
You May Also Like –
« AWS vs Azure vs Google Cloud – Features & Pricing Comparison How to Serve WordPress Pages on Subdomains[without plugin] »